To create an object-oriented quiz game using Ruby, you will need to do the following:
Define the quiz game class:
Start by creating a class for the quiz game. This class should have a constructor method that initializes the quiz game with a list of questions and answers. You can also add methods to the class to start the quiz, display a question, and check the answer.
Define the question class:
Next, create a class for the questions. This class should have a constructor method that initializes the question with a prompt and a list of possible answers. You can also add a method to the class to display the question and another method to check the answer.
Create the quiz game:
To create an instance of the quiz game, you will need to create an array of question objects and pass it to the quiz game constructor. You can then call the start method on the quiz game to start the quiz.
Display the questions:
To display the questions, you can use a loop to iterate over the list of questions and call the display method on each question. You can also use the gets method to get the user’s answer and pass it to the check_answer method to check if it is correct.
Keep track of the score:
To keep track of the score, you can add a score variable to the quiz game class and increment it whenever the user answers a question correctly. You can also add a finish method to the quiz game class to display the final score.
Here is an example of what the quiz game class might look like:
Related post: How to Fine-Tune your Rails application with Rails Service Objects
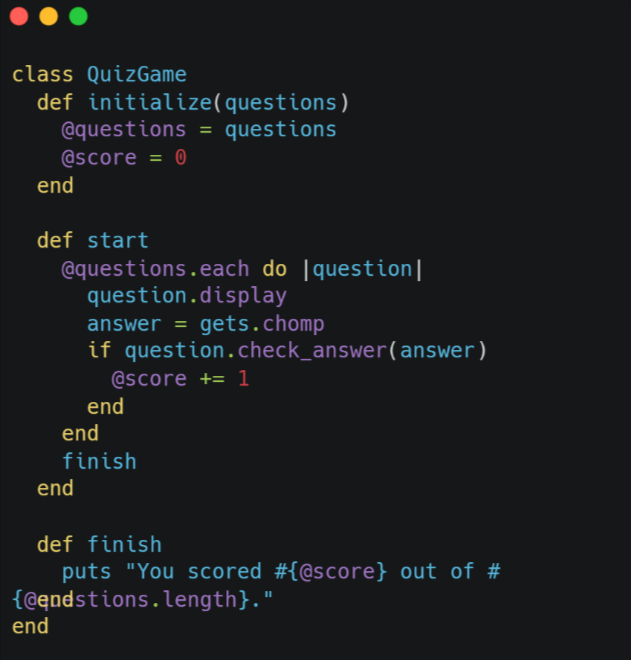
And here is an example of what the question class might look like:
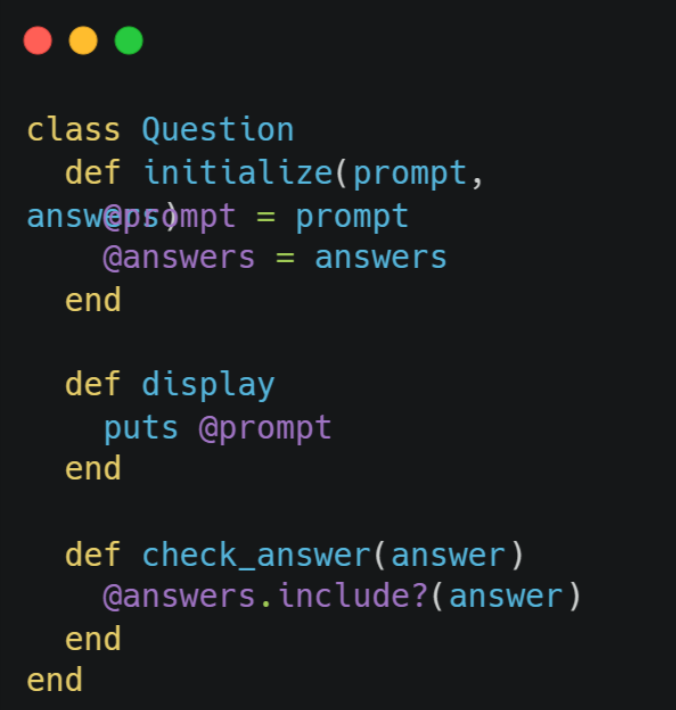
To create an instance of the quiz game and start it, you can do something like this:
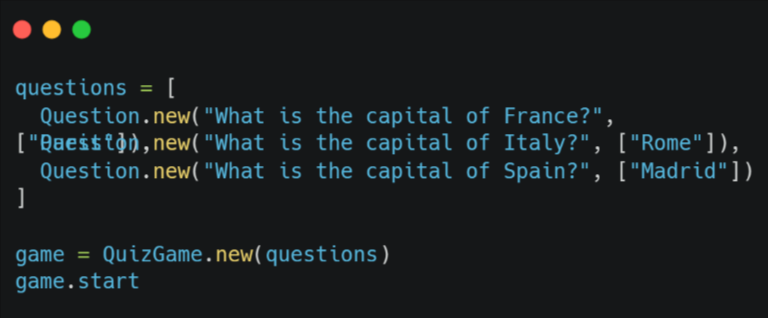
That’s a basic overview of how to create an object-oriented quiz game using Ruby. You can add more features and complexity to the game by adding more methods and variables to the classes and by using more advanced features of the Ruby language. Ruby on Rails microservices can help to improve scalability, maintainability, and flexibility and promotes faster deployment.
Leave a Reply