Ruby is an interpreted programming language. This basically means that Ruby source code is not pre-compiled like it is with languages like C or C++, but rather is compiled and executed at run time. One advantage of being an interpreted language is that we can type Ruby code directly into the interpreter and have it run interactively and in real-time. This is an excellent way to learn Ruby and experiment with different code structures.
The ‘irb’ tool is used to enter interactive Ruby code. We already have ‘irb’ installed if we are running Windows and have installed Ruby using the one-click installer. If we’re using Linux, it’s possible that ‘irb’ isn’t yet installed.
We will check the installation by doing the following:
irb -v
If the ‘irb’ tool is not installed, then install it using the ‘sudo apt-get install irb’ command. After installing irb, run it as follows:
$ irb
It will redirect to the following:
irb(main):001:0>
We will then enter the codes of Ruby to run from here. For printing a simple output from the terminal, we will use the following command:

We can also perform arithmetic operations directly from the terminal. When we press the Enter key, whatever we type at the ‘irb’ prompt is executed.
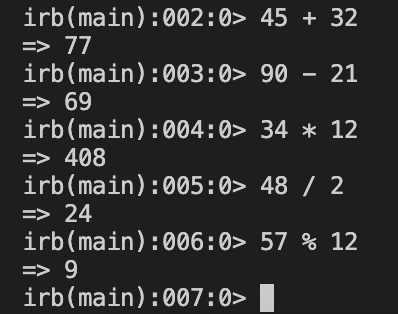
We will then look at an example where we will assign variables:
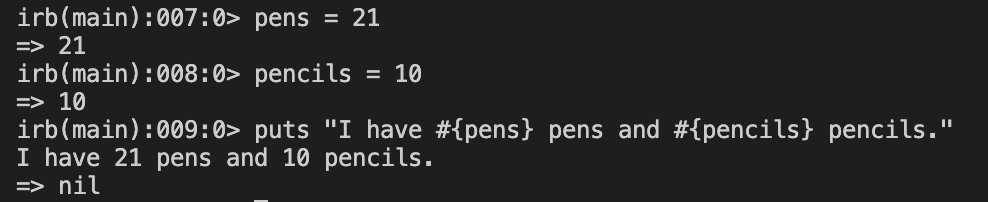
Example for taking input from the user:

The function ‘gets.chomp’ is used to accept user input.
To print without a new line, use ‘print’ instead of ‘puts’.
The function ‘gets.chomp.to i’ is used to obtain integer input from the user.
The function ‘gets.chomp.to_f’ is used to obtain float (decimal) input from the user.
These are the most fundamental concepts of simple ruby examples to learn Ruby that are crucial for new Ruby programmers.
[NOTE: Use ‘CTRL+D’ to exit from the ‘irb’ prompt.]
Leave a Reply