It is typically the best practice in Rails to keep the majority of your business logic and execution within your models, and thus away from your controllers. That isn’t to say the paradigm of developing “fat models” is necessarily appropriate in all cases, and of course, your design pattern should be based on the needs of the project.
Yet, at the very least, the concept of tiny controllers (and by extension, fewer objects passed to your views) is a good practice to get into. To achieve this, you need look no further than using a facade pattern, which effectively provides a higher-level interface that can aggregate important functionality and pass that interface along to lesser interfaces.
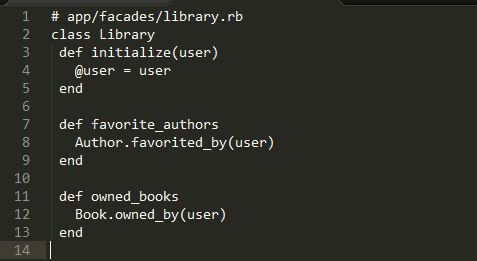
In the case of Rails, this means creating a facade class that exposes a variety of methods and objects needed in a particular view, without requiring the controller to directly access all of these methods and objects individually.
Here’s a simple example of using a facade in our Book Dojo application:
A basic example, but here we have the Library class with acts as a facade to gather information about the current user along with his or her favorite authors and owned books.
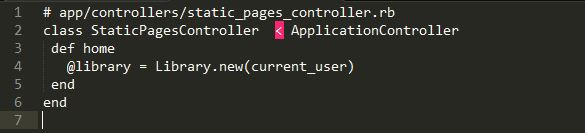

We can then simply pass that information along to the controller that will make use of it with only one single instance object, @library.
Now, the view of the home page that renders this also needs only to receive one instance object of @library, and can invoke the methods therein to get the appropriate information.
While this is a simple example, it should give you an idea of how a facade pattern can clean up your controllers and views considerably.
Leave a Reply