Since OpenStreetMap is the biggest and editable free geographic database and project, the API is free of charge. To geolocate a single address, you just have to give the email of your OSM account as input to the Nominatim
class, instead of the API key:
from geopy.geocoders import Nominatim geolocator = Nominatim(user_agent="your_email") location = geolocator.geocode(address1) print(location) # None
OpenAI
Unfortunately, the location returned by OpenStreetMap API is None because it doesn’t recognize the address.
Indeed, this API struggles to locate most of the street addresses in the dataset because it doesn’t have good address coverage in all the areas.
For this reason, we need to create another field unique_address_osm with less information:
l_cols_concat = ['City','State','Country'] df['unique_address_osm'] = df['Street Address'].str.cat(others=df[l_cols_concat], sep=',',na_rep='') address1_osm = df['unique_address_osm'].iloc[0]
OpenAI
After, we pass the new address, and we try again to extract the latitude and the longitude from the street address:
address1_osm = df['unique_address_osm'].iloc[0] location = geolocator.geocode(address1_osm) print('Latitude: '+str(location.latitude)+', Longitude: '+str(location.longitude))
OpenAI

As before, we apply the service_geocode()
function to all the column unique_address_osm:
df['LAT_LON_osm'] = df['unique_address_osm'].apply(lambda x: service_geocode(geolocator,x)) df[['unique_address_osm','LAT_LON','LAT_LON_osm']].head()
OpenAI
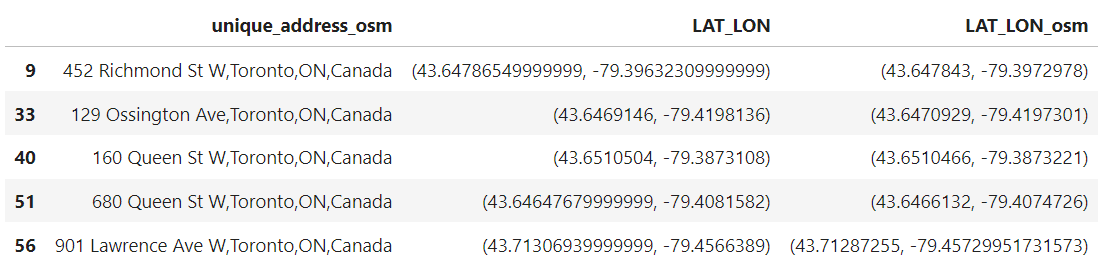
Although we didn’t specify the name of the museum, OpenStreetMap returns very similar coordinates to GoogleMapsAPI.
Leave a Reply